
In general, GNU is more "open" than ANSI and provides more syntax support.
#Memcpy borland c++ code#
In the actual program design, in order to meet the ANSI standard and improve the portability of the program, we can write the code to achieve the same function as follows: void * pvoid (char *)pvoid++ //ANSI: correct GNU: correct ( char *)pvoid += 1 //ANSI: Error GNU: Correct GNU and ANSI have some differences. Therefore, the following statements are correct in the GNU compiler: pvoid++ //GNU: correct pvoid += 1 //GNU: correct pvoid++ results in an increase of 1. But the famous GNU (abbreviation of GNU's Not Unix) does not think so, it specifies the arithmetic operation of void * is consistent with char *. According to the ANSI (American National Standards Institute) standard, you cannot perform arithmetic operations on void pointers, that is, the following operations are illegal: void * pvoid pvoid++ //ANSI: error pvoid += 1 //ANSI: Wrong//The ANSI standard recognizes this because it insists: the pointer for arithmetic operations must be sure to know the size of the data type it points to.//For example: int *pint pint++ //ANSI: The result of correct pint++ is to increase sizeof(int). Rule 3: Use the void pointer type carefully. Therefore, whether in C or C++, if the function does not accept any parameters, you must specify that the parameters are void. In C++, you cannot pass any parameters to a function without parameters, and the error message "'fun': function does not take 1 parameters" appears. For example: add (int a, int b) compiles correctly and outputs 1, which means that in C language, you can give functions without parameters Transmit any type of parameters, but compiling the same code in a C++ compiler will cause an error. But many programmers mistake it for the void type. In the C language, any function without a return value type limitation will be used by the compiler as Return integer value processing. Use of void The rules for using the void keyword are given below: Rule 1: If the function does not return a value, it should be declared as void type. The following statement compiles with errors: void *p1 int *p2 p2 = p1 prompt "'=': cannot convert from'void *'to'int *'". We can say that "men and women are both humans", but we cannot say that "people are men" or "people are women".
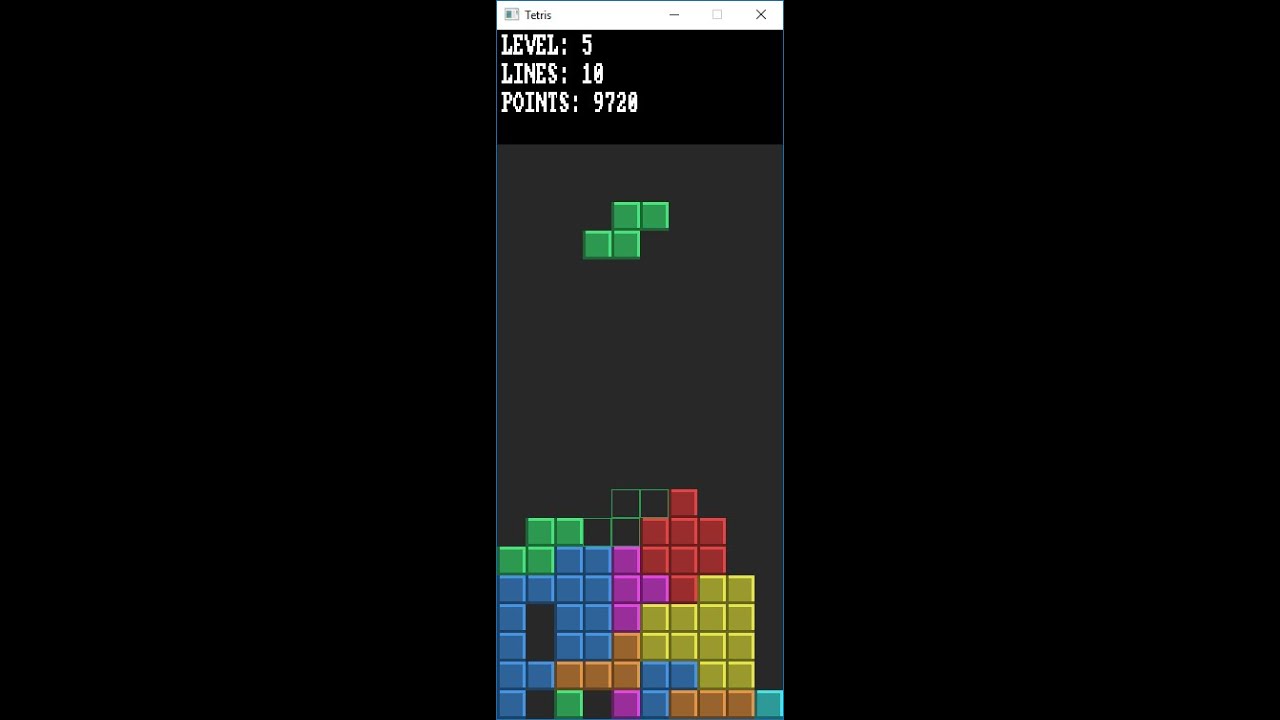
Because "no type" can contain "typed", and "typed" cannot contain "no type". For example: float *p1 int *p2 p1 = p2 where p1 = p2 statement will compile error, prompt "'=': cannot convert from'int *'to'float *'", must be changed to: p1 = ( float *)p2 and void * is different, any type of pointer can be directly assigned to it, without mandatory type conversion: void *p1 int *p2 p1 = p2 But this does not mean that void * also Can be assigned to other types of pointers without casting. As we all know, if the pointers p1 and p2 are of the same type, then we can directly assign values between p1 and p2 if p1 and p2 point to different data types, we must use the cast operator to convert the pointer type on the right side of the assignment operator Is the type of the left pointer.
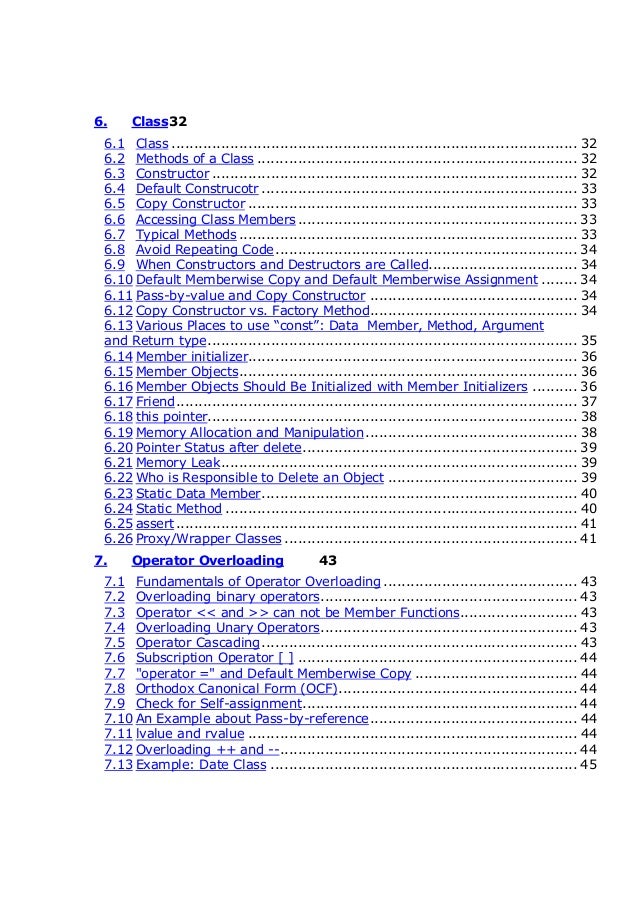
We will elaborate on the above two points in Section 3.

The real function of void is: (1) The limitation of function return (2) The limitation of function parameters. However, even if the compilation of void a does not go wrong, it has no practical meaning. Void almost only has the function of "comment" and restricting the program, because no one has ever defined a void variable, let us try to define: void a This line of statement will make an error when compiling, prompting "illegal use of type'void'". The meaning of void Void literally means "no type", void * is "no type pointer", void * can point to any type of data. This article will explain the profound meaning of the void keyword, and detail the use methods and techniques of void and void pointer types. Overview Many beginners do not understand the void and void pointer types in the C/C++ language very well, so there are some errors in their use.
